Python is a powerful high-level programming language that has been gaining popularity for building all kinds of applications. With its easy-to-read syntax and extensive libraries, Python makes app development efficient, maintainable, and cross-platform compatible.
Python was first released in 1991 by developer Guido van Rossum. Since then, it has become one of the most widely used programming languages in the world. Python is often praised for its simplicity, versatility, and large supportive community.
Python holds a prominent place among the most widely adopted programming languages, supported by an extensive and active developer community. According to 2023 research conducted by Statista, around 48% of these developers choose Python to build stable and dependable backends for applications.
The broad acceptance of this language can be attributed to its easily understandable syntax, user-friendly simplicity, and generous offering of free Python libraries that contribute to enhancing productivity and efficiency among developers.
👉🏾 Other Swiftspeed Users Also Read: How to Create an AI App Using OpenAI’s API – Comprehensive Guide
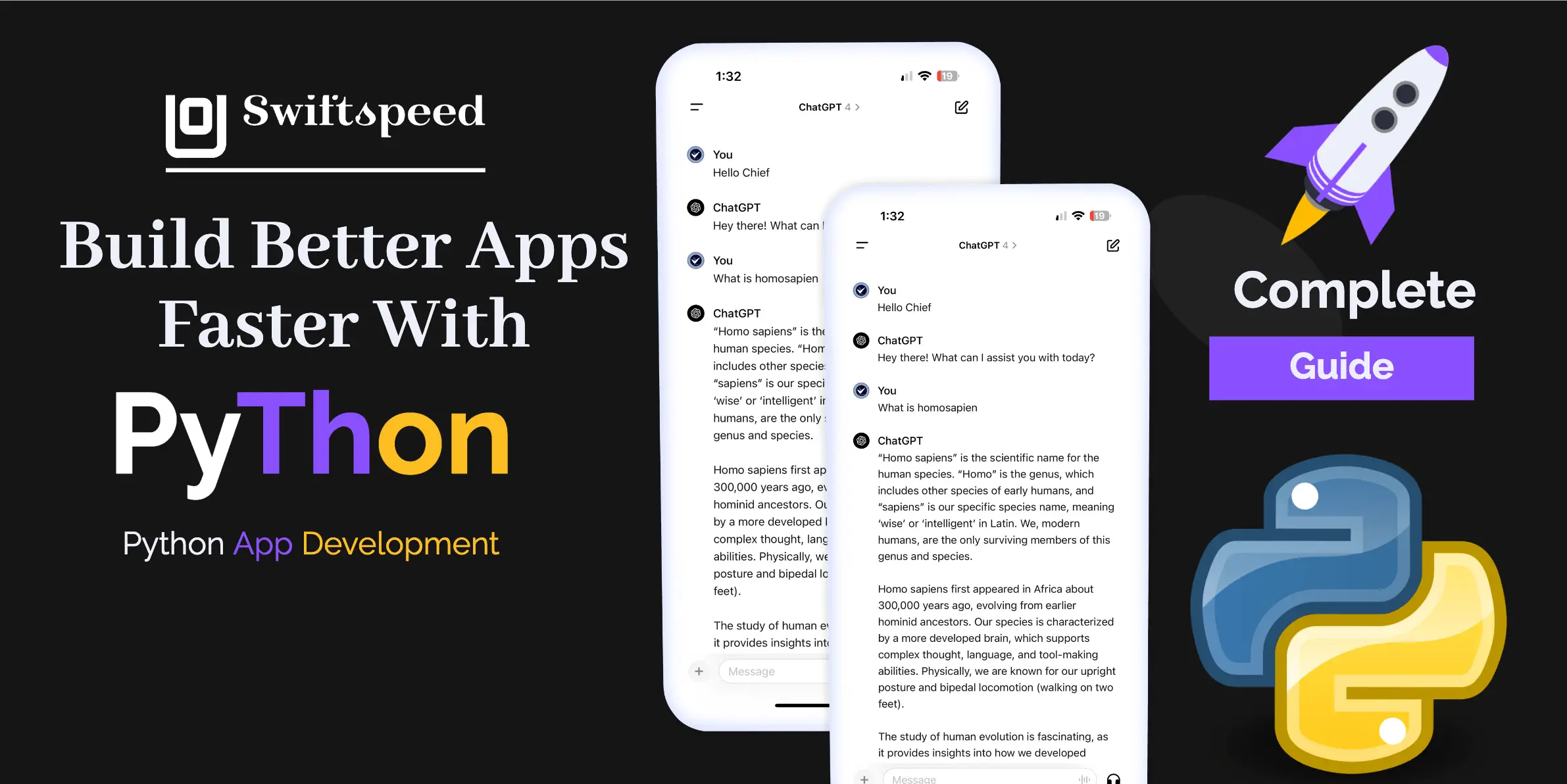
Python’s Popularity In App Development
Python has become one of the most popular programming languages for building all types of applications, from simple scripts to complex web and mobile apps. Here are some key reasons why Python is widely used in app development:
- Python consistently ranks as one of the top 3 most popular languages on coding sites like Stack Overflow. In Stack Overflow’s 2020 survey, Python was the 2nd most loved language behind Rust, showing its popularity among developers.
- Python is easy for beginners to learn thanks to its simple syntax that resembles everyday English. This makes it a great first language for new programmers to pick up.
- Python has a large, active, open-source community supporting it. This community provides many high-quality libraries and frameworks for app development in Python.
- Python works very well for prototyping and creating MVPs quickly. The fast development cycle makes it ideal for startups and small teams.
- Python can be used to build all types of apps – web apps with frameworks like Django and Flask, mobile apps on Android and iOS using tools like Kivy, and desktop GUI apps using Tkinter or PyQt.
- Python is versatile enough to build anything from simple scripts to machine learning models and large scalable web services. Major companies like Google, Facebook, Netflix, Uber, and Reddit use Python for their back-end infrastructure.
With these advantages, it’s no wonder Python has become a go-to choice for creating all types of applications – from simple scripts to complex web and mobile apps. Python provides the capabilities, tools, and support that developers need for app projects.
For app development, Python offers many advantages:
- Simple and Easy to Learn: Python has a very readable, almost English-like syntax with less verbose code compared to other languages. This makes Python easy to learn and great for beginners. At the same time, Python is powerful enough for large, complex app projects.
- Cross-Platform Compatibility: A major benefit of Python is its seamless cross-platform support. Python apps built for one operating system, such as Windows, can often run on another, like macOS or Linux, with minimal porting required.
- Extensive Libraries and Frameworks: The Python Package Index (PyPI) contains over 200,000 Python packages and modules for diverse use cases. For app developers, Python has many specialized web, mobile, and desktop frameworks available.
- Supports Multiple Programming Paradigms: Python is a multi-paradigm language, meaning Python code can be structured in different styles, including object-oriented, procedural, and functional programming. This flexibility allows developers to use the approach that works best.
- Improved Productivity and Maintenance: Python’s high level of abstraction allows developers to accomplish more in fewer lines of code compared to lower-level languages. Python code is often more readable and modular, making apps faster to develop and easier to maintain.
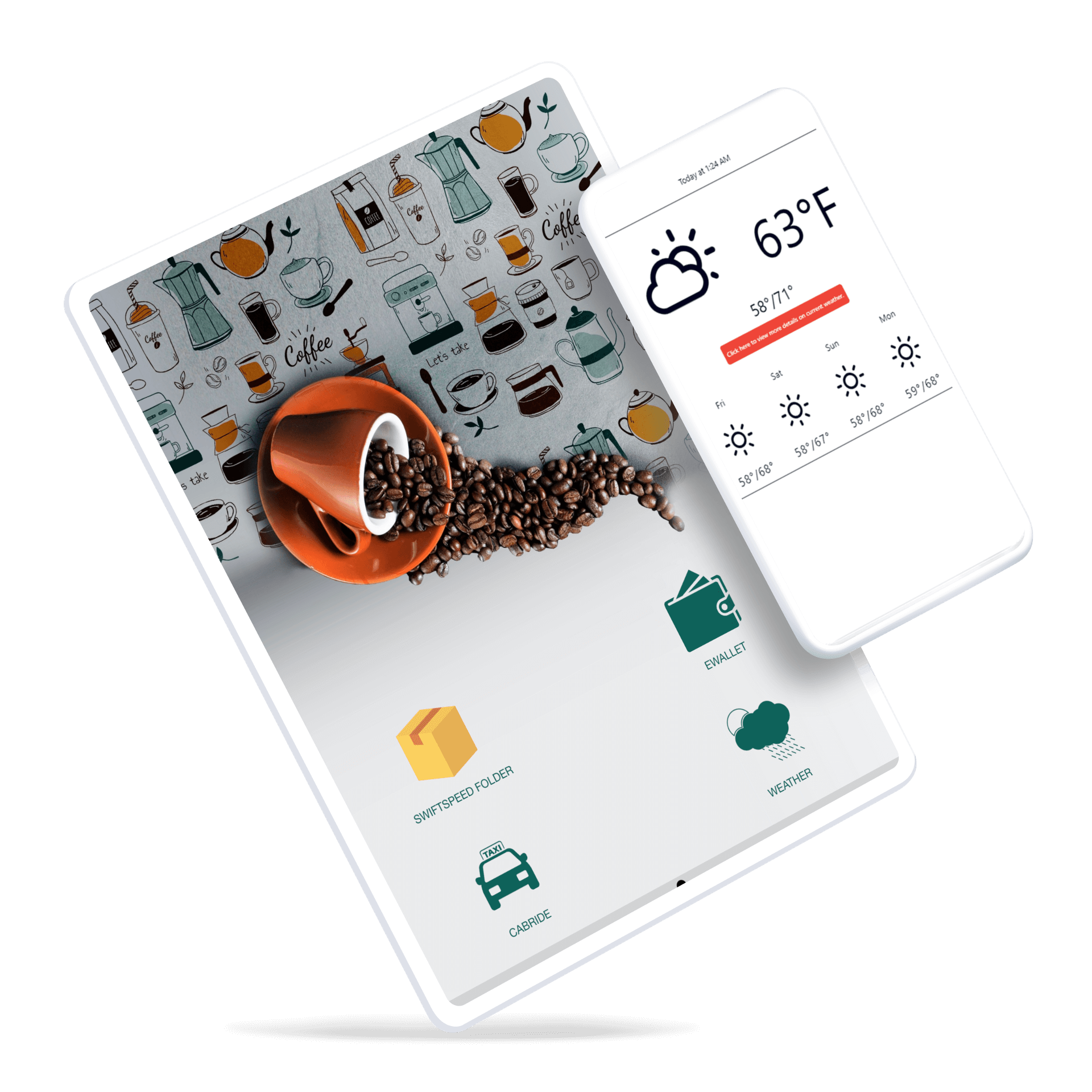
Python Frameworks for App Development
Python has several popular web frameworks that make it easy to build web applications. Here are some of the most widely used:
Django
Django is a batteries-included web framework that provides many built-in features for web development, including an ORM, templating engine, admin interface, and more.
Pros:
- Full-featured and versatile for building complex, database-driven websites
- Fast and secure
- Scales well for high-traffic sites
- Extensive documentation and community support
Cons:
- Can feel heavyweight for smaller applications
- Steeper learning curve than some frameworks
Django is a great choice for content management systems, news/media sites, social platforms, e-commerce, and other data-intensive web apps.
Flask
Flask is a lightweight framework that keeps the core simple but extensible.
Pros:
- Minimalist and flexible
- Easy to get started
- Scales well for complex apps
Cons:
- The lack of built-in features means more extensions needed
- The loose structure requires more decisions from the developer.
Flask works well for building APIs, web services, simple websites, and web apps that don’t require extensive backend administration. We wrote extensive documentation on building an AI app using Python Flask here.
Pyramid
Pyramid is built on top of WSGI (Web Server Gateway Interface) and uses a modular architecture.
Pros:
- Flexible and extensible
- Can choose components a la carte
- Large ecosystem of add-ons
Cons:
- Relatively new, smaller community
- Not as many learning resources as Django
Pyramid is a good choice for data-driven web apps that require flexibility. The modular architecture makes it adaptable.
The major Python web frameworks each have strengths and weaknesses. Django is the most full-featured, Flask is minimalist, and Pyramid is extremely flexible. Consider the needs of the application when choosing a framework.
Developing Web Apps with Python
Python is commonly used for web application development thanks to its batteries-included philosophy. The standard library contains modules like Flask, Django, and FastAPI that make it straightforward to get started building web apps in Python.
To develop a web app in Python, the general steps are:
- Set up a Python virtual environment and install dependencies like Flask or Django.
- Create the basic directory structure for the web app. This usually includes folders for templates, static files, application code, etc.
- Write the main application code. This will include routes, views, models, forms, etc, depending on the framework.
- Create templates for dynamic content generation. HTML templates can render data from views with Flask’s Jinja or Django’s templating engine.
- Configure settings like the development server host and port, database connections, secret keys, etc.
- Run the development server and access the app locally to test and develop further.
- Add CSS, JavaScript, images, and other static assets.
- Implement user authentication, databases, API integration, payment systems, etc., as needed.
- Deploy the web app to a remote production server. Popular Python app deployment options include Docker, Heroku, AWS, and custom servers.
For example, here is a simple web app built with Flask that displays “Hello World!”:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return 'Hello World!'
if __name__ == '__main__':
app.run()
This demonstrates a basic Flask app routing structure. More complex apps can be built by adding templates, models, forms, and other components. Python makes it easy to incrementally develop and iterate on web apps.
Pause for a moment; you don’t know how to code in Python or any other app development languages but would still like to build your app? Explore our app builder software today and create cross-platform mobile apps without writing a single line of code, easier, cheaper, faster, and better👇🏽
Build Android and iOS Apps with Swiftspeed
Create premium apps without writing a single line of code, thanks to our user-friendly app builder. Build an app for your website or business with ease.
Developing Mobile Apps with Python
Python is a popular language for developing mobile apps, particularly for iOS and Android. Here are some of the top options for building mobile apps with Python:
Kivy
Kivy is an open-source Python library designed for rapid mobile app development. It can be used to build apps for iOS, Android, Windows, Linux, and macOS. Kivy provides tools for creating the graphical interface and supports multitouch input.
To get started with Kivy:
- Install Kivy through pip or conda.
- Create a .py file and import Kivy modules like Button, Label, etc.
- Design the app UI by creating a layout with Kivy widgets.
- Add event handlers to respond to touch input and control app logic.
- Package the app with Buildozer to generate Android and iOS binaries.
A simple Kivy app can be built in just a few dozen lines of Python code. The framework abstracts away much of the low-level mobile development complexity.
BeeWare
BeeWare provides a collection of tools for building native Python apps across multiple platforms, including mobile. Key components include:
- Briefcase – handles project generation and app packaging
- Toga – provides GUI toolkit and widgets
- Rubicon-OS – converts Python to native code
To build a mobile app with BeeWare:
- Configure a Briefcase project.
- Design the interface with Toga.
- Add platform support for iOS and Android.
- Use Briefcase to generate platform projects and app packages.
BeeWare enables native performance while using Python end-to-end. The learning curve is steeper than a framework like Kivy.
Pythonista
Pythonista is an iOS-exclusive app that allows Python programming and execution on iPhones/iPads. It includes an editor, a Python interpreter, and access to iOS APIs.
With Pythonista, developers can write Python scripts that interact with iOS, creating simple utility apps and prototypes without needing Xcode. It makes an easy on-ramp for iOS development with Python.
Finished scripts can be turned into full apps with additional Xcode work. Many find Pythonista a handy tool for testing snippets and ideas on-device.
Distribution
For mobile app distribution, Kivy and BeeWare apps can be uploaded to the Apple App Store and Google Play Store like any other native app.
Pythonista scripts can be distributed through the app itself or require additional Xcode processing to publish standalone. There are restrictions on the types of apps permitted without a full Apple review.
Overall, Python enables many options for building and delivering mobile applications on major platforms. With the right frameworks and tools, Python can be used to develop full-featured mobile apps.
👉🏾 Other Swiftspeed Users Also Read: Complete list of top mobile app stores in 2024
Developing Desktop Apps with Python
Python is a versatile language that can also be used to build desktop GUI applications. There are several GUI frameworks available for Python that make it easy to create desktop apps with Python.
Tkinter
Tkinter is Python’s standard GUI library that comes bundled with Python installations. It provides a simple and easy way to build desktop apps using the Tkinter widgets.
To build a desktop app in Tkinter, you first import the Tkinter module and create a root window. You can then add Tkinter widgets like buttons, labels, text boxes, etc, to build your GUI. Tkinter supports features like events and bindings that allow you to define interactions.
With just a few lines of code, you can create a simple desktop app GUI. Tkinter is straightforward to use and ideal for building basic desktop applications in Python.
PyQt
PyQt is a popular Python binding for the Qt framework. It provides a more powerful and flexible GUI framework compared to Tkinter. PyQt offers a wide range of widgets and comes with many customization options for building sophisticated UIs.
PyQt integrates well with other Python libraries and tools. It is well-suited for building complex desktop applications in Python. The PyQt framework follows an event-driven programming paradigm which makes the code more maintainable.
To build a desktop GUI app in PyQt, you design the visual interface using Qt Designer. Then you add the Python logic by importing the GUI file and connecting event handlers to the UI elements. PyQt supports features like signals, slots, and events to enable interactions in the GUI.
Distributing Python Desktop Apps
To distribute your Python desktop application, you need to bundle it into a standard executable file. Some options for distributing Python desktop apps include:
- PyInstaller – Can bundle Python apps into stand-alone executables for distribution across platforms.
- cx_Freeze – Converts Python programs into executables with all their dependencies included.
- Briefcase – A tool to publish Python desktop apps for multiple platforms from a single codebase.
- Py2app – For freezing Python scripts into macOS applications.
By using these tools, you can package your Python desktop application into a standard executable file. This executable can be distributed to end users without requiring them to install Python or any other dependencies.
Key Python Modules and Libraries
Python comes with a vast collection of powerful modules and libraries that make app development faster and easier. These modules provide reusable code for common programming tasks, so developers don’t have to reinvent the wheel. Here are some of the most essential Python modules for app development:
Requests
Requests is a simple yet powerful HTTP library for Python. It allows developers to send HTTP requests extremely easily. Requests can get or post HTML pages, JSON APIs, files, and imagery using just a few lines of code. The simple API and built-in JSON decoding make Requests perfect for web scraping and integrating with web services.
Pandas
Pandas is a fast and efficient data analysis library for Python. It provides high-performance structures like DataFrames and Series to make data cleaning and analysis easy. Pandas is great for data manipulation tasks like combining, filtering, grouping, and reshaping data sets. For app developers, Pandas is ideal for loading and analyzing input data.
NumPy
NumPy adds support for large multi-dimensional arrays and matrices to Python. It includes mathematical and logical operations on arrays, making it efficient for numerical computing. NumPy is the foundation for data science and machine learning in Python. For app developers, NumPy enables high-performance mathematical operations for tasks like image processing, linear algebra, and data visualization.
Matplotlib
Matplotlib is Python’s most popular 2D plotting library. It can generate publication-quality figures and graphs with just a few lines of code. Developers can use Matplotlib to visualize data, create interactive plots, and add imagery to apps. From bar charts to heatmaps, Matplotlib has a wide range of plotting capabilities for app developers.
Tkinter
Tkinter provides Python with a cross-platform GUI toolkit for building desktop apps. It comes bundled with Python and provides widgets like buttons, menus, and dialog boxes. Tkinter has a simple API and provides a native look and feel on Windows, Mac, and Linux. For developers building Python desktop apps, Tkinter is the standard GUI framework.
By leveraging these powerful Python libraries, developers can build robust apps efficiently with less code. The wide range of functionality provided by Python’s modules is one of the reasons it has become so popular for app development.
Debugging and Testing Python Apps
Python provides powerful tools for debugging and testing apps during development. Here are some key techniques:
Debugging
- Use the built-in
pdb
module to set breakpoints and step through code. This allows inspection of variables and execution flow. - Implement logging using the
logging
module. Log key info, warnings, and errors to debug issues and trace execution flow. - Use an IDE like PyCharm, which has excellent debugging support through breakpoints, variable watches, and more.
- Leverage debuggers like
IPython
,pudb
, or commercial solutions like JetBrains PyCharm for debugging.
Testing
- Write unit tests using the
unittest
framework that comes built-in with Python. Structure code so units can be independently tested. - Consider test-driven development with
unittest
to drive design through testing. Write tests first, then implement code to make tests pass. - For more advanced testing, use
pytest
which supports fixtures, code coverage reporting, and parametrized testing. - Use mocking libraries like
unittest.mock
orpytest-mock
to isolate units under test from dependencies.
Tips
- Use
try/except
blocks to gracefully handle errors and prevent crashes. Log errors to diagnose issues. - Refactor code for readability and modularity. Break code into logical units that are easier to test and debug.
- Validate input data and add checks for edge cases that could cause unexpected behavior.
- Enable warnings and linters to catch issues early, like undefined variables, unused imports, etc.
- Use static-type checkers
mypy
to detect type errors and inconsistencies. - Profile performance with
cProfile
libraries likeline_profiler
to isolate and optimize bottlenecks.
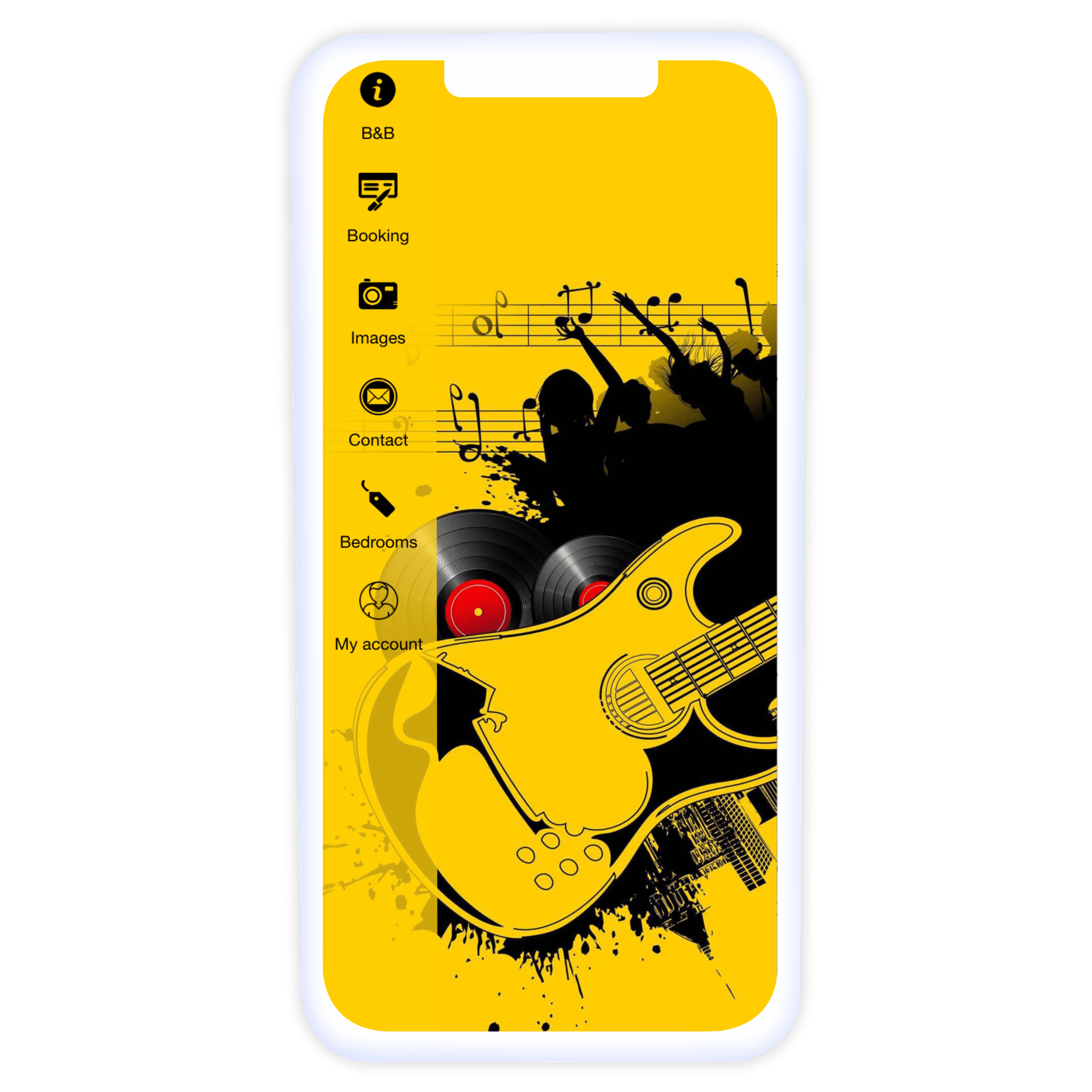
Deploying Python Apps
Python apps can be deployed to various platforms and cloud providers. Here are some of the most popular options:
Heroku
Heroku is a platform-as-a-service (PaaS) that makes deploying Python apps easy. To deploy to Heroku:
- Create a Heroku account and install the Heroku CLI.
- Make sure your app has a requirements.txt file with app dependencies.
- Login to Heroku using the CLI and create a new app:
heroku create my-app-name
- Add a Procfile to tell Heroku how to run your app. For a Flask app it would contain:
web: gunicorn app:app
- Push your code to Heroku to deploy:
git push heroku master
Heroku will install dependencies and start your app. You can easily scale the dynos and resources for your app as needed.
AWS Elastic Beanstalk
Elastic Beanstalk makes deploying Python apps on AWS infrastructure easy. Steps to deploy:
- Sign up for AWS and create an Elastic Beanstalk application.
- Configure your Python environment by selecting a platform branch.
- Upload your code as a ZIP file or connect your repository.
- Elastic Beanstalk will handle installing dependencies, configuring instances, load balancing, auto-scaling, and monitoring.
You have full control over the underlying EC2 instances, auto-scaling, load balancers, etc. Elastic Beanstalk simplifies infrastructure management.
👉🏾 Other Swiftspeed Users Also Read: The Ultimate Guide to iPhone Screen Resolutions and Sizes in 2024
Managing Infrastructure
Most cloud platforms make it easy to scale your app infrastructure up and down. Scaling can be configured based on metrics like CPU usage, requests per second, or custom CloudWatch alarms.
Cloud platforms also include tools for monitoring your apps in production. You can view metrics, logs, and trace errors back to their source. Many provide alerting systems to notify you of issues.
With the right architecture, Python apps can scale seamlessly. Technologies like message queues and memcached can help. Auto-scaling groups ensure capacity matches demand.
Conclusion
Python has emerged as one of the most popular programming languages for app development due to its ease of use, flexibility, vast library support, and large active community.
In this article, we explored some of the key ways Python is used for web, mobile, and desktop application development. We looked at popular frameworks like Django, Flask, Kivy, and PyQt that make it simpler to build fully-featured apps. We covered important Python modules like Tkinter, SQLite, and sockets that provide the building blocks for many Python programs.
Though Python may not always be the absolute best choice for every use case, it provides an excellent combination of productivity, performance, scalability, and ecosystem support for most app development needs.
As Python continues to grow in popularity, we can expect more tools and resources to emerge that further simplify app creation. The future is bright for using Python to build anything from simple scripts to complex applications.
For those interested in leveraging Python for their own apps, there are many free tutorials, books, and Python courses available to help you get started. The Python community is welcoming to beginners and seasoned developers alike. Don’t be afraid to jump in and start building.
With its versatility and growing adoption across fields like data science, web development, DevOps, and finance, knowledge of Python is a valuable skill set for any programmer’s toolkit. Learning Python for app development is an investment that will pay dividends for years to come.