Commonly recognized as a programming choice for creating apps on .NET
Framework for more than two decades, VB.NET
has served to build Windows desktop and web solutions. With Microsoft’s new emphasis on cloud services and cross-platform capabilities, many VB.NET developers want to transform their applications using .NET
Core.
As an open-source and cross-platform version of .NET
, .NET
Core runs successfully on Windows, Linux and macOS. By shifting to .NET
Core from VB.NET
applications, developers gain improved performance and access to new functionality.
We will discuss essential motives for moving VB.NET
applications into .NET
Core, along with illustrating the migration process in this thorough guide.
Benefits of Migrating VB.NET Apps to .NET Core
Here are some of the major benefits you can expect from the modernization of your VB.NET
applications to .NET
Core:
Enhanced Performance
.NET
Core has been optimized for speed and memory usage compared to the .NET
Framework. You can expect up to 60% faster startup times for .NET
Core applications. The runtime and libraries have also been streamlined, allowing .NET
Core apps to perform faster execution of code.
Cross-Platform Support
One of the biggest advantages of .NET
Core is its support for multiple platforms, including Windows, macOS, and Linux. By migrating apps to .NET
Core, you can deploy them on the platform of choice without needing separate code bases.
👉🏾 Other Swiftspeed Users also read: A Complete Guide to Mobile App Development in 2024
Improved Scalability
.NET
Core offers improved scalability capabilities for building and running large cloud-based apps. The runtime has improved support for microservices architecture, containerization and orchestration platforms like Docker and Kubernetes.
Access to Latest Features
Microsoft prioritizes quickly upgrading .NET
Core by releasing new versions every few months. When moving their apps to .NET
Core, developers can benefit from the fresh innovations and enhancements found in the framework.
Future Proof Code
A clear message from Microsoft indicates that .NET
Core will lead the way for .NET
applications, while the .NET
Framework will slowly be discontinued. By shifting to .NET
Core VB.NET
will remain preserved for years ahead.
Consistent Improvements
Each new version of .NET
Core enhances the runtime and libraries, prioritizing better performance and reliability enhancements. Staying informed is simple for VB.NET
professionals.
Modernizing Your Application for Improved Performance
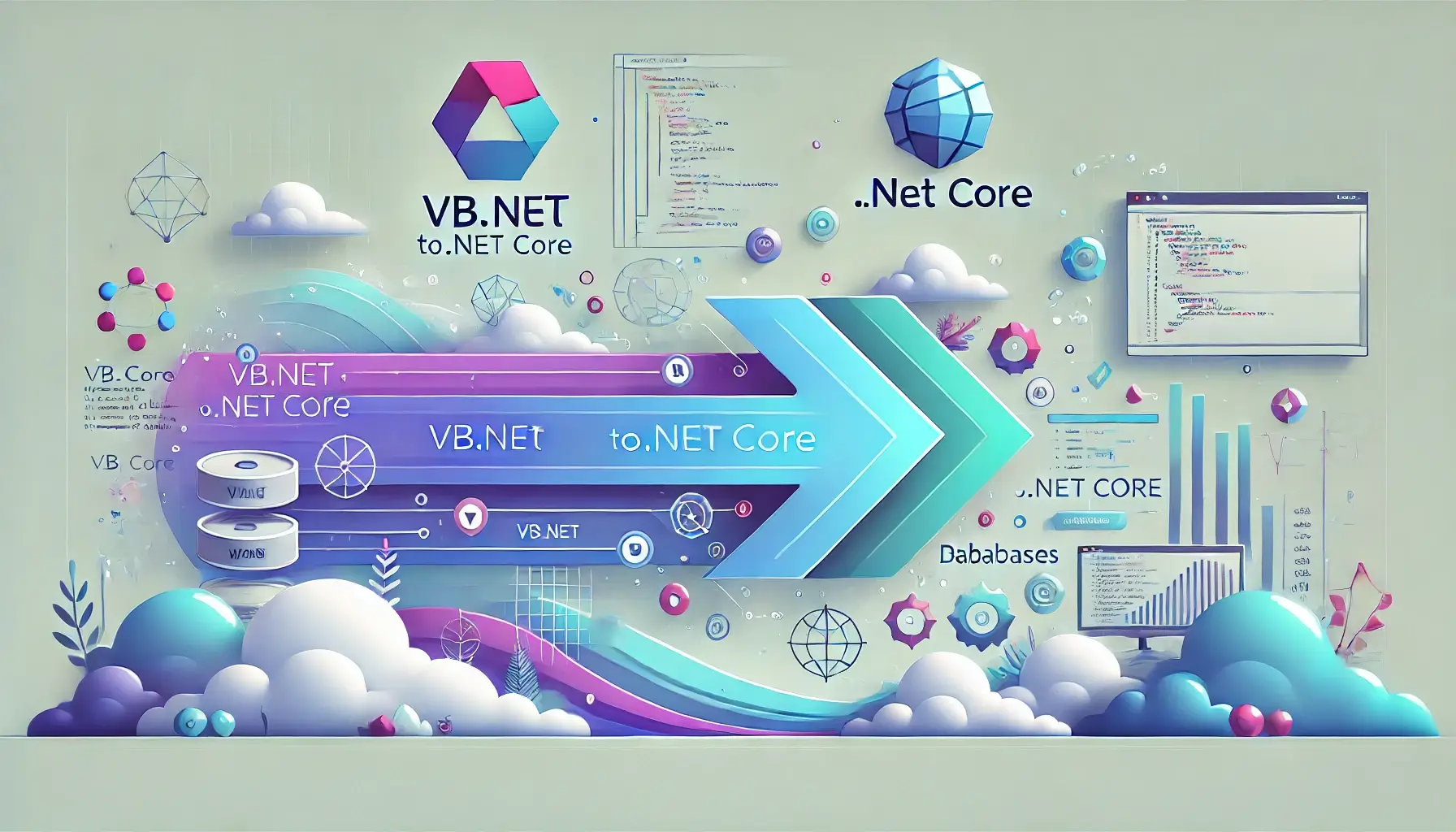
Migrating VB.NET
desktop applications to .NET
Core involves the following key steps:
1. Analyze Existing Application
First, analyze the VB.NET application to grasp all the dependencies used by its code. Capture the system design along with the referenced frameworks and libraries.
2. Install Required Tools
Install Visual Studio 2022 and .NET
6 SDK on development machines. Also, install .NET
Portability Analyzer to scan compatibility issues.
3. Run .NET
Portability Analyzer
Use the .NET
Portability Analyzer tool to scan the VB.NET app and uncover code areas that are not compatible with .NET Core 3.1/5.0/6.0.
Also, find feature gaps.
4. Create .NET
Core Project
Create a new .NET
Core app project in Visual Studio. The project structure will be different from VB.NET
Framework apps. Configure project accordingly.
5. Migrate Code Files
Migrate all VB.NET
code files from the old project to the new.NET
Core project. Refactor code to replace .NET
Framework namespaces and APIs with corresponding .NET
Core namespaces and APIs.
6. Migrate Application Configuration
VB.NET apps use app.config
files for configuration. These need to be migrated to the appsettings.json structure in .NET
Core.
7. Migrate Data Access Layer
Data access code interacting with databases needs review. Replace old ADO.NET
data access code with newer approaches like Dapper for .NET
Core.
8. Migrate
UI Layer (Optional)
For desktop applications with UI layer, it can optionally be migrated to cross-platform UIs like AvaloniaUI or Uno Platform for Windows/Linux/macOS support.
9. Setup Dependencies
The existing .NET
Framework dependencies used by the project will not work. Identify replacements for them that are compatible with .NET
Core and set them up.
10. Refactor As Needed
Based on best practices for .NET
Core, refactor other parts of code as needed – e.g. logging, caching, background workers. Leverage native DI container.
11. Test End-to-End
Rigorously test the migrated application end-to-end on all functionality, UI flows, data layers etc. Fix issues until app stability is proven on .NET
Core.
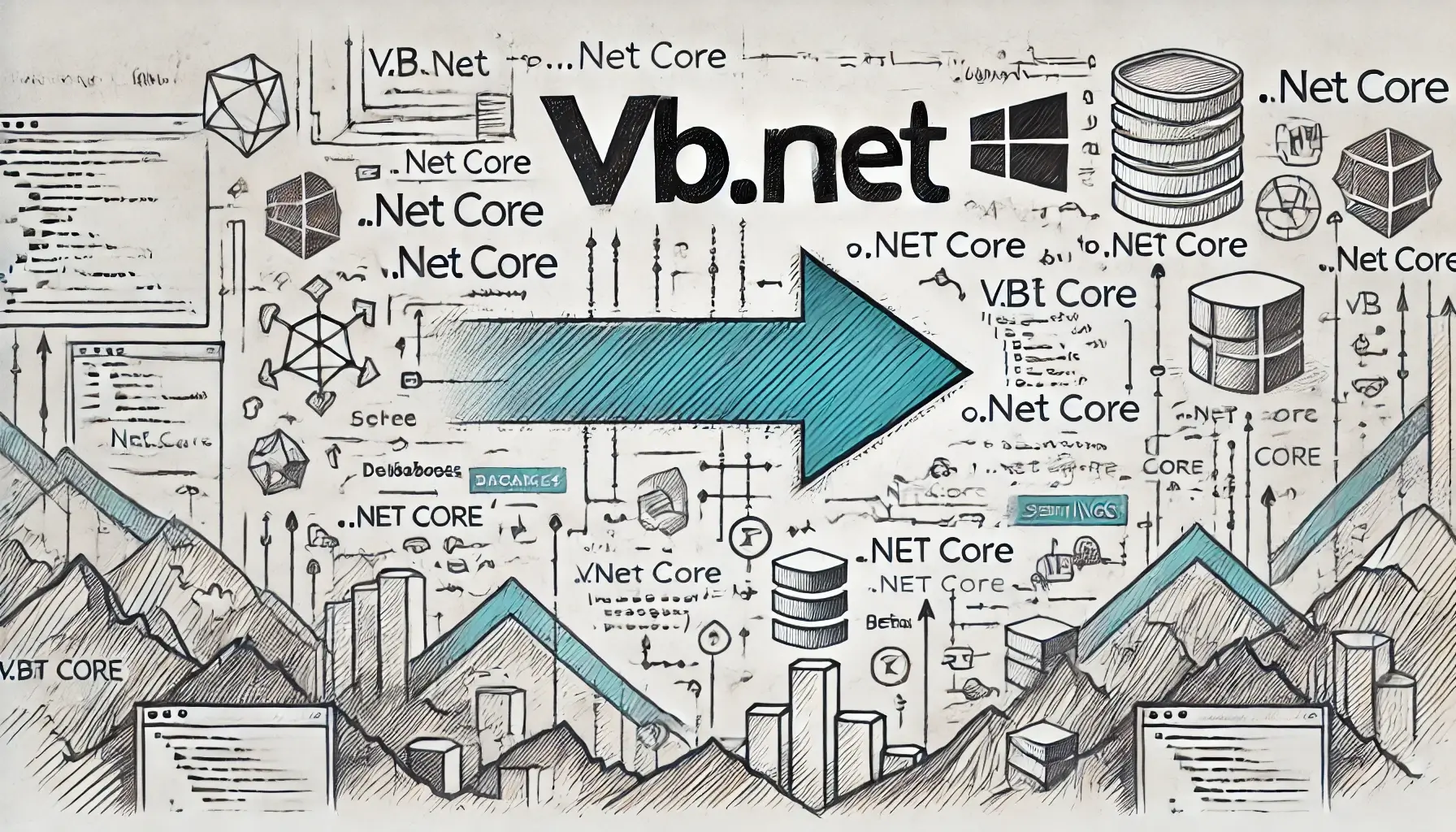
Step-by-Step Migration Process
Now let us explore some of the key migration steps explained above in more technical detail:
Analyzing Existing Application
When starting the migration, developers should invest time to understand and document the existing VB.NET
application’s thoroughly:
- Overall architecture: Class library projects, console apps, Windows services etc. and how they interact with each other
- UI layer: Windows Forms? WPF? ASP.NET WebForms? Used frameworks/libraries?
- Middle tier and business logic: Design patterns used? Domain model constructs?
- Data access layer:
ADO.NET
? Entity Framework? External databases connected? - Application configurations: How is app.config used? Connection strings? App settings?
- External integrations: Any direct connections to external systems? Protocols/technologies used?
- Dependencies: Full list of NuGet packages and their purposes
- Platform-specific code: Any Windows-specific code used? Registry access? Special folders?
👉🏾 Other Swiftspeed Users also read: How to make money from apps Using Fremium app monetization
Such an analysis is crucial so that equivalent capabilities can be mapped in .NET
Core during migration.
Upgrading IDE and Tools
VB.NET
apps need to be re-developed on Visual Studio 2022 to target .NET Core 3.1
or later versions. Older IDEs do not include templates and tooling required for .NET
Core.
Install the latest .NET
6 SDK for access to all the .NET
Core templates, project structure guidance and tooling needed to build applications using C#, VB.NET or F# targeting .NET
Core.
The .NET Portability Analyzer should also be installed to scan the existing portfolio of VB.NET
assemblies and uncover compatibility issues early.
Understanding Project Structure
The project structure used for .NET
Framework and .NET
Core applications is quite different:
VB.NET .NET Framework
- Uses
.vbproj
project files - Has Global Assembly Cache (GAC)
- Uses
\bin
folder for builds
.NET Core
- Uses new SDK-style
.vbproj
project format - No more concept of GAC
The migration process should focus on moving code files into appropriate folders as per the modular structure above.
Migrating Code Files
Once the new .NET
Core project is setup, the next step is to migrate all the individual VB.NET
code files from old project to this new project.
The code itself will need refactoring to:
- Replace usage of
.NET
Framework namespaces with new.NET
Core namespaces - Update code that uses any deprecated
.NET
Framework APIs - Leverage newer APIs available only in
.NET
Core
This may require changes across models, data access code, utility functions, integration code etc.
Replacing Configuration Files
Another key task is the migration of configuration files from the app.config
structure used in .NET Framework over to the new appsettings.json file based configuration approach used in .NET
Core.
Settings include:
- Connection strings
- Application settings
- Logging configuration
- External service endpoints
Here is an example appsettings.json file with common settings:
{
"ConnectionStrings": {
"MyDB": "Server=localhost;Database=MyAppDB;User Id=sa;"
},
"Logging": {
"LogLevel": {
"Default": "Warning"
}
},
"AppSettings": {
"ServiceURL": "https://api.myservice.com",
"AnotherKey": "AnotherValue"
}
}
The code needs to be updated to leverage Microsoft.Extensions.Configuration namespace to read these settings.
Refactoring Data Access Code
The data access code interacting with databases using old ADO.NET
approaches will need refactoring to use newer methods like Dapper for .NET
Core.
Here is an example of how a data access class can leverage Dapper to query a database in .NET
Core:
Imports System.Data.SqlClient
Imports Dapper
Public Class MyRepository
Private readonly IConfiguration _config
Public Sub New(configuration As IConfiguration)
_config = configuration
End Sub
Public Function GetData() As List(Of MyObject)
Using conn As New SqlConnection(_config.GetConnectionString("MyDB"))
Dim results = conn.Query(Of MyObject)("SELECT * FROM MyTable")
Return results.ToList()
End Using
End Function
End Class
Dapper provides high performance data access combined with simplicity and flexibility to interact with relational databases.
Testing Thoroughly
Due to the extent of changes needed across various application layers during VB.NET
migration to .NET
Core, thorough end-to-end testing is vital to ensure all use cases function correctly.
Teams should plan out extensive test plans across:
- Unit testing of individual components
- Integration testing capabilities
- End-to-end workflows
- Functional validation
- Performance benchmarking
- Security reviews
Plan out appropriate test data coverage and reports to establish confidence in quality before going live.
Conclusion
Migrating VB.NET
desktop and web applications to.NET
Core requires careful planning, code refactoring, and testing to ensure applications retain full functionality. While the process poses challenges, the benefits of enhanced performance, cross-platform support, easier scalability and leveraging the latest Microsoft innovations make it worthwhile.
Thorough testing across units, integrations, UI flows and performance benchmarking is vital before going live. With its faster execution times and lower resource usage, .NET
Core empowers VB.NET
applications for the future while retaining existing code investments. As Microsoft invests heavily in growing the .NET
Core ecosystem, migrating applications early on future-proofs them while realizing material gains.